Certainly! In SwiftUI, if you want to programmatically scroll a List
to a specific position, you can use the ScrollViewReader
. This provides a scrollTo()
method that allows you to move to any row inside the list by providing its ID and optionally an anchor12.
Here’s how you can achieve this:
import SwiftUI
struct ContentView: View {
@StateObject private var scrollingProxy = ListScrollingProxy()
var body: some View {
VStack {
HStack {
Button(action: { self.scrollingProxy.scrollTo(.top) }) {
Image(systemName: "arrow.up.to.line")
.padding(.horizontal)
}
Button(action: { self.scrollingProxy.scrollTo(.end) }) {
Image(systemName: "arrow.down.to.line")
.padding(.horizontal)
}
}
Divider()
List(0..<200, id: \.self) { item in
Text("Item \(item)")
.id(item)
Divider()
}
.background(ScrollViewReader { sp in
ScrollView {
LazyVStack {
ForEach(0..<200, id: \.self) { item in
VStack(alignment: .leading) {
Text("Item \(item)").id(item)
Divider()
}
.frame(maxWidth: .infinity)
.padding(.horizontal)
}
}
.onReceive(self.scrollingProxy.$direction) { action in
guard !items.isEmpty else { return }
withAnimation {
switch action {
case .top:
sp.scrollTo(items.first!, anchor: .top)
case .end:
sp.scrollTo(items.last!, anchor: .bottom)
default:
return
}
}
}
}
})
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In this example, the List
is wrapped in a ScrollViewReader
, and the scrollTo()
method is used to scroll to the top or bottom of the list based on the user’s button taps. Adjust the content and customize the buttons as needed for your specific use case.
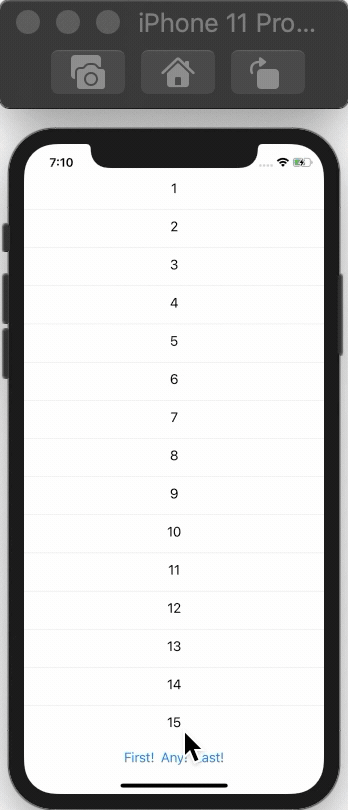
Remember to test thoroughly and adapt this approach to your specific requirements. Happy coding!