Setup Apple Pay in iOS Xcode
Apple Pay tutorial swift 5
set up Apple Pay :Apple Pay is form of payment integration special used in iOS devices especially in iPhone
Since making your own NFC payment framework wouldn’t go over excessively well with Apple, this instructional exercise will rather see how to coordinate Apple Pay into your own iOS applications. This is not quite the same as in-application buys for advanced substance — Apple Pay allows you to offer products to your clients from inside an application.
Apple pay integration in swift 5
Here is the apple pay developer documentation.
In this Apple Pay instructional exercise, you’ll figure out how to compose an application that allows clients to purchase truly cool instructional exercises and stuff utilizing only the comfort of their fingerprints
Integrate apple pay in iOS App
Initially before integrating please Apple pay support for the countries
https://support.apple.com/en-us/HT207957
Also check the Apple Pay UI Guidelines :
https://developer.apple.com/design/human-interface-guidelines/apple-pay/overview/buttons-and-marks/
here are the steps to integrate apple pay in iOS app
How we can setup the integration of Apple Pay in our application
- Create a Merchant ID
- Configure Apple Pay capabilities in Xcode for your project
- Create a sandbox user in iTunes Connect
- Add a test card
- Create a payment request in your project
- Handle the result
1. Create a Merchant ID
Head over to http://developer.apple.com.Go to the member Center and click on certificate, Identifier & ProfileIdentifierApp ID’s
Initially create the merchant ID in Apple Developer Account. This ID allows the apple to identify the app as a merchant for the payment with respective your bundle ID.
Click on the plus Icon
In the particular Description ,Add the name of the app. In identifier, type “merchant” + the bundle identifier.
Example : “merchant.com.navya.E-Store”
we need to get the certificate signing request . Open up Keychain Access, and from the top menu select “Certificate Assistance” and “Request a Certificate from a Certificate Authority” as shown in the picture.
We’re almost back to coding! We just need to create a payment processing certificate, which will be used to secure transaction data. Go back to the member center and select “All Certificates” from the side menu. Tap the plus “+” button on the top right corner to create a new certificate
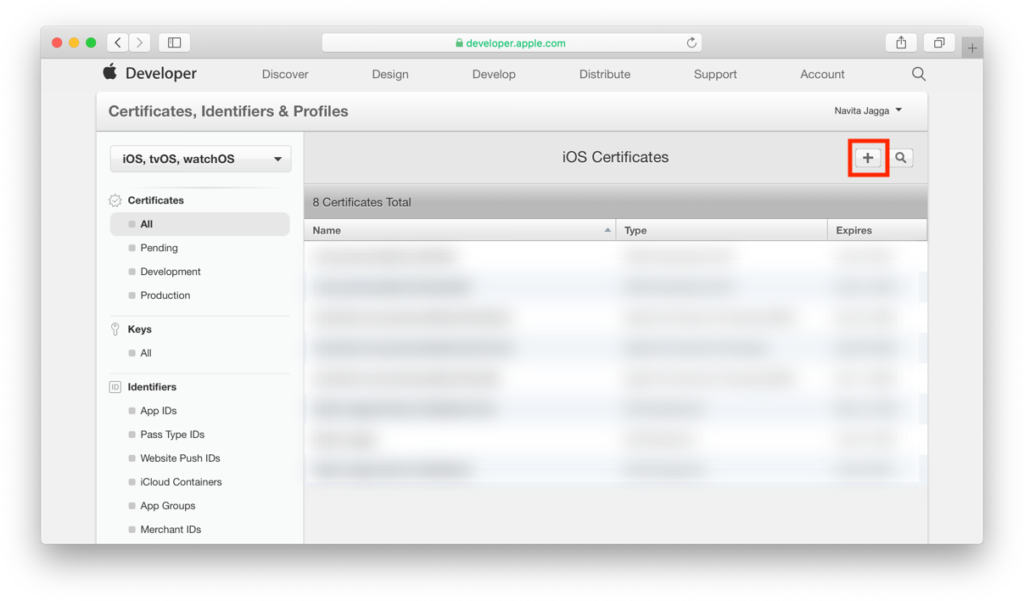
Under “Production”, select “Apple Pay Payment Processing Certificate”.

Then, select the merchant ID you created earlier. Next, under “Apple Pay Payment Processing Certificate”, tap on “Create Certificate”. Apple will ask, “Will payments associated with this Merchant ID be processed exclusively in China?” Select yes. Continue through the process, and it will ask you to upload your CSR that you saved from Keychain Access.
Download it and save it with your project. Then, double click on it, and it will be processed in Keychain Access.
2. Configure Apple Pay capabilities in Xcode for your project
Open Xcode Under capabilities enable apple pay and add the correct merchant ID’s
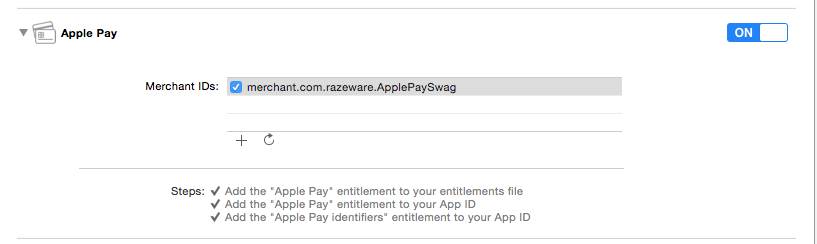
Creating a Payment Request
Open PaymentViewController.swift and add the following import to the top of the file:
apple pay integration swift 5
Now we can configure our payment request
import PassKit
For this we use delegate PKPaymentAuthorizationViewControllerDelegate
In there mainly 2 methods we use for ApplePay
func paymentAuthorizationViewControllerDidFinish(_ controller: PKPaymentAuthorizationViewController) ----> Tells the delegate that payment authorization finished.
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didAuthorizePayment payment: PKPayment, handler completion: @escaping (PKPaymentAuthorizationResult) -> Void) ----> Tells the delegate that the user has authorized the payment request.
–Code View for SetUp ApplePay–
extension ViewController : PKPaymentAuthorizationViewControllerDelegate{
func purchaseItem(currencyCode : String, billAmount : Double) {
let request = PKPaymentRequest()
request.merchantIdentifier = "merchant.com.navya.E-Store”
request.supportedNetworks = [PKPaymentNetwork.visa, PKPaymentNetwork.masterCard, PKPaymentNetwork.amex]
request.merchantCapabilities = PKMerchantCapability.capability3DS
request.countryCode = "USD"
request.currencyCode = currencyCode
request.paymentSummaryItems = [
PKPaymentSummaryItem(label: "nayva", amount: NSDecimalNumber(value: billAmount)) ---> In there you can Add Owner Name
]
let applePayController = PKPaymentAuthorizationViewController(paymentRequest: request)
applePayController?.delegate = self
if PKPaymentAuthorizationViewController.canMakePayments( usingNetworks: request.supportedNetworks){
self.present(applePayController!, animated: true, completion: nil)
}
else{
print("Apple Pay not available for device")
}
}
//Mark- It shows that Apple Pay supported or not.
func applePaySupported() -> Bool {
return PKPaymentAuthorizationViewController.canMakePayments() && PKPaymentAuthorizationViewController.canMakePayments(usingNetworks: [.amex, .visa, .masterCard])
}
//Mark- On the Basis of Payment_data you can find card type
func cardTypeSetUp(value : Int)-> String{
switch value {
case 1:
return "DBCRD"
case 2:
return "CRDC"
default:
return ""
}
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didSelect shippingMethod: PKShippingMethod, handler completion: @escaping (PKPaymentRequestShippingMethodUpdate) -> Void){
print(shippingMethod)
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didSelectShippingContact contact: PKContact, handler completion: @escaping (PKPaymentRequestShippingContactUpdate) -> Void){
print(contact)
}
func paymentAuthorizationViewControllerDidFinish(_ controller: PKPaymentAuthorizationViewController) {
controller.dismiss(animated: true, completion: nil)
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController, didAuthorizePayment payment: PKPayment, handler completion: @escaping (PKPaymentAuthorizationResult) -> Void) {
completion(PKPaymentAuthorizationResult(status: PKPaymentAuthorizationStatus.success, errors: []))
Card_type = cardTypeSetUp(value: Int(payment.token.paymentMethod.type.rawValue))
Card_name = payment.token.paymentMethod.network?.rawValue ?? ""
appleCard_Type = payment.token.paymentMethod.network?.rawValue ?? ""
payment_Data = payment.token.paymentData.base64EncodedString()
switch PKPaymentAuthorizationStatus.success {
case .success:
break
default:
break
}
}
}
Add the apple pay logo : For apple pay logo reference you can check to apple documentation for set up Apple Pay
We’re finished! Run the app, and you’ll see the payment view controller
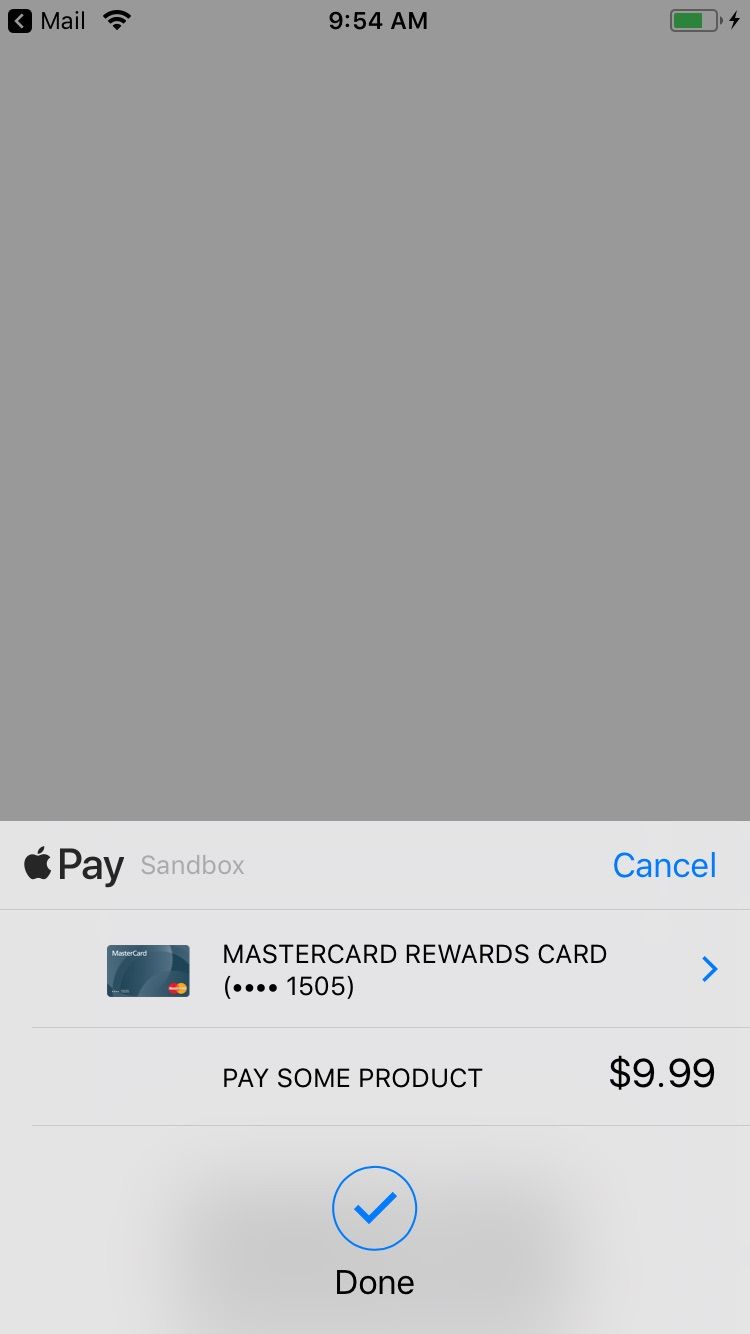